- SalesforceChaCha
- Posts
- 💃 Design Patterns for Salesforce🕺
💃 Design Patterns for Salesforce🕺
Off-load object instantiation to a Factory
Good morning, Salesforce Nerds! Imagine you’re running a factory.
But this isn’t just any factory - it’s a factory of ideas, of ingenious objects that serve your Salesforce org like loyal minions. 🦹
Each one has a unique purpose:
👉️ Sending emails
👉️ Delivering SMS messages
👉️ Firing off push notifications.
How do you ensure this factory doesn’t become a chaotic mess of tangled blueprints and overlapping designs? 🥴
This is where the Factory Method design pattern shines! ✨ Your blueprint for orderly, scalable, and elegant object creation.
Let’s dive into what makes this pattern a must-know for any Apex developer and how you can wield it to build better solutions. 👍️

TABLE OF CONTENTS
Design Patterns for Salesforce
THE FACTORY WHAT?
WHAT IS IT?
The Factory Method is a creational design pattern that acts like the Willy Wonka of object creation. 🎩
Instead of hardcoding which type of object to create, it leaves the decision to a dedicated factory method.
Allowing the client code to stay blissfully ignorant of what goes on behind the scenes. 😁
Awesome … so why should you care?
🔥 It keeps your code flexible and ready to embrace change.
🔥 It adheres to the Open-Closed Principle, which is a fancy way of saying, “You can add new stuff without breaking the old stuff.”
🔥 It’s perfect for situations where the exact type of object needed isn’t known until runtime.
HOW DOES IT COME TOGETHER?
THE CORE INGREDIENTS
Before we start churning out objects, let’s break down the key components of the Factory Method pattern:
🏭️ The Factory (a.k.a Creator): This is the mastermind. It contains the logic to decide which object to create.
📜 The Product Interface: This defines what all products have in common. Think of it as the contract every product must honor.
📦️ Concrete Products: These are the specific classes that implement the product interface, each with its own unique flavor.
🧑💻 Client Code: This is the consumer of the factory. It asks for an object but doesn’t concern itself with how that object is created.
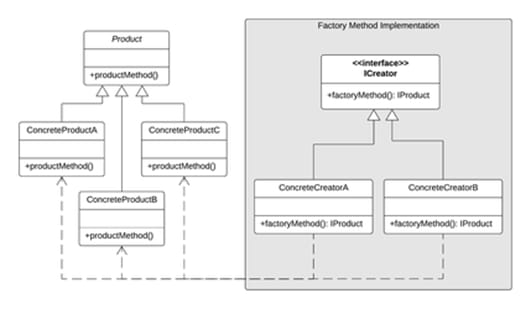
Generalized UML for the Factory Pattern
BRINGING ALL TOGETHER
SHOW ME THE CODE!
Time revisit the opening scenario.
As a reminder, imagine you’re tasked with sending different types of notifications 👇️
Email, SMS, and Push notifications.
Here’s how the Factory Method design pattern saves the day 🦸
1️⃣ Step 1: Define the Product Interface
public interface INotification {
void send(String message);
}
This interface ensures every notification type has a send
method. It’s the promise that all notifications will play by the same rules.
2️⃣ Step 2: Create Concrete Products
public class EmailNotification implements INotification {
public void send(String message) {
System.debug('Email sent: ' + message);
}
}
public class SMSNotification implements INotification {
public void send(String message) {
System.debug('SMS sent: ' + message);
}
}
public class PushNotification implements INotification {
public void send(String message) {
System.debug('Push notification sent: ' + message);
}
}
Each of these classes brings its own flair to the notification game.
3️⃣ Step 3: Build the Factory
public class NotificationFactory {
public static INotification getNotification(String type) {
if (type == 'Email') return new EmailNotification();
if (type == 'SMS') return new SMSNotification();
if (type == 'Push') return new PushNotification();
throw new IllegalArgumentException('Unknown notification type: ' + type);
}
}
The factory method decides which concrete product to create based on input. It’s like a chef preparing a dish based on your order.
4️⃣ Step 4: Use the Factory in Client Code
INotification notification = NotificationFactory.getNotification('Email');
notification.send('Hello, Factory Method!');
And voilà! Your client code gets a ready-to-use object without knowing the nitty-gritty of its creation!
THE GLORIOUS BENEFITS
WHY IT’S AWESOME
The Factory Method design pattern isn’t just fancy jargon; it’s a game-changer for Apex developers.
Here’s why:
🩰 Flexibility: Adding new types of notifications? Just create a new class and tweak the factory. No need to touch the client code.
🎁 Maintainability: All the instantiation logic lives in one place (the factory), making your code easier to read and maintain.
📈 Scalability: Whether you’re adding one new notification type or ten, the pattern scales effortlessly.
Some common scenarios where the Factory Method shines:
⚡️ Dynamic Object Creation: Need to create objects based on runtime data? This pattern’s got you covered.
🔁 Integration Layers: Use it to handle different external service implementations through a unified interface.
🏗️ Separation of Concerns: Keep your controllers or triggers focused on logic, while the factory handles object creation.
Pro-tips!
🤏 Start Small: Practice with simple examples like notifications or report generators.
📜 Leverage Interfaces: Ensure all your products implement a common interface for maximum flexibility.
🤗 Embrace Static Methods: In Salesforce, static factory methods are a convenient and widely accepted approach.
WRAPPING IT UP
CONCLUSION
The Factory Method design pattern is your ticket to crafting scalable, maintainable, and flexible Apex code. 🎟️
It’s like having a personal assistant who knows exactly which object to create and when.
So, whether you’re building a notification system, integrating external services, or just trying to make your code less of a spaghetti monster 🍝 , the Factory Method is here to save the day.
Now go forth, Salesforce professional, and build your object factory like the Apex pro you were meant to be! 🤓
SOUL FOOD
Today’s Principle
"Always code as if the person who ends up maintaining your code will be a violent psychopath who knows where you live."
and now....Salesforce Memes



What did you think about today's newsletter? |